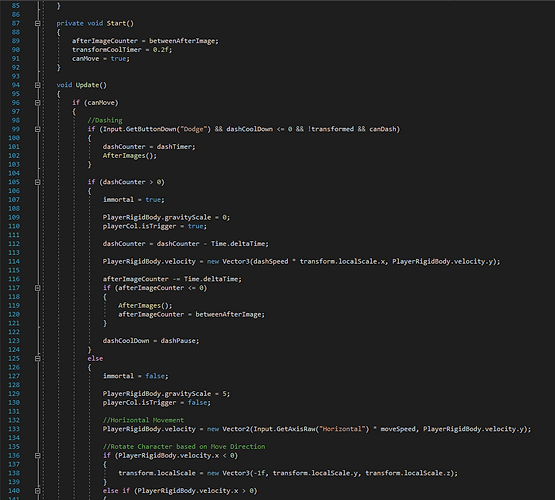
Code Library
Player Control
public class PlayerController : MonoBehaviour { public Rigidbody2D PlayerRigidBody; public AbilityTracker abilities; public float moveSpeed; public float jumpForce; public Transform groundPoint; public LayerMask groundLayer; private bool isGrounded; public Animator playerAnim; public BulletController shotFire; public Transform pointOfFire; public Transform bombPoint; public GameObject bomb; public float bombCoolTime; [HideInInspector] public float bombCooler; public bool bombCooled = false; private bool canDoubbleJump; public bool doubleJumpUnlock = false; public int extraJumpCount; private int extraJumps; private int jumpCounts; public float dashSpeed; public float dashTimer; public float dashCounter; public bool canDash = false; public CapsuleCollider2D playerCol; public SpriteRenderer playerSR; public SpriteRenderer afterImageSR; public float afterImageLife; public float betweenAfterImage; private float afterImageCounter; public Color afterImageColor; public float dashPause; public float dashCoolDown; public GameObject standingPlayer; public GameObject transformedPlayer; public float transformTime; public float transformTimer; public float transformCooldown; public float transformCoolTimer; public bool transformCooled; public bool transformed = false; public Animator transformedAnim; public bool immortal = false; public bool canMove; public static PlayerController instance; private void Awake() { if (instance != null) { Destroy(gameObject); } else { instance = this; DontDestroyOnLoad(gameObject); } if (doubleJumpUnlock) { extraJumpCount = 1; } else { extraJumpCount = 0; } } private void Start() { afterImageCounter = betweenAfterImage; transformCoolTimer = 0.2f; canMove = true; } void Update() { if (canMove) { //Dashing if (Input.GetButtonDown("Dodge") && dashCoolDown 0) { immortal = true; PlayerRigidBody.gravityScale = 0; playerCol.isTrigger = true; dashCounter = dashCounter - Time.deltaTime; PlayerRigidBody.velocity = new Vector3(dashSpeed * transform.localScale.x, PlayerRigidBody.velocity.y); afterImageCounter -= Time.deltaTime; if (afterImageCounter <= 0) { AfterImages(); afterImageCounter = betweenAfterImage; } dashCoolDown = dashPause; } else { immortal = false; PlayerRigidBody.gravityScale = 5; playerCol.isTrigger = false; //Horizontal Movement PlayerRigidBody.velocity = new Vector2(Input.GetAxisRaw("Horizontal") * moveSpeed, PlayerRigidBody.velocity.y); //Rotate Character based on Move Direction if (PlayerRigidBody.velocity.x 0) { transform.localScale = Vector3.one; } } if (dashCoolDown > 0) { dashCoolDown -= Time.deltaTime; Color tempColor = AbilityTracker.instance.dashGrayLayer.color; tempColor.a = 0.6f; AbilityTracker.instance.dashGrayLayer.color = tempColor; AbilityTracker.instance.dashGrayLayer.fillAmount = 0 + dashCoolDown / dashPause; if (transformed) { AbilityTracker.instance.dashGrayLayer.fillAmount = 1; } } //Check Ground isGrounded = Physics2D.OverlapCircle(groundPoint.position, 0.2f, groundLayer); if (isGrounded) { extraJumps = extraJumpCount; jumpCounts = 0; } else { jumpCounts = 1; } //Jumping if (Input.GetButtonDown("Jump") && (isGrounded || canDoubbleJump)) { if (isGrounded && extraJumps >= 0 && doubleJumpUnlock) { canDoubbleJump = true; } else if (isGrounded || extraJumps = 0 && doubleJumpUnlock) { playerAnim.SetTrigger("DoubleJump"); } if (canDoubbleJump || !doubleJumpUnlock) { PlayerRigidBody.velocity = new Vector2(PlayerRigidBody.velocity.x, jumpForce); extraJumps -= 1; } } //Shooting if (Input.GetButtonDown("Fire1") && !transformed) { Instantiate(shotFire, pointOfFire.position, pointOfFire.rotation).moveDir = new Vector2(transform.localScale.x, 0); playerAnim.SetTrigger("Shooting"); } else if (Input.GetButtonDown("Fire1") && transformed && bombCooled) { Instantiate(bomb, bombPoint.position, bombPoint.rotation); bombCooled = false; bombCooler = bombCoolTime; } if (bombCooler > 0 && !bombCooled) { bombCooler -= Time.deltaTime; Color tempColor = AbilityTracker.instance.bombGrayLayer.color; tempColor.a = 0.6f; AbilityTracker.instance.bombGrayLayer.color = tempColor; AbilityTracker.instance.bombGrayLayer.fillAmount = bombCooler / bombCoolTime; if (bombCooler = transformTime) { standingPlayer.SetActive(true); transformedPlayer.SetActive(false); transformed = false; transformCoolTimer = transformCooldown; transformCooled = false; AbilityTracker.instance.AbilityUpdate(); transformTimer = 0; } } else if (Input.GetButtonUp("Fire3")) { transformTimer = 0; Color tempColor = AbilityTracker.instance.transformGrayLayer.color; tempColor.a = 0f; AbilityTracker.instance.transformGrayLayer.color = tempColor; } } else if (!transformed && transformCooled) { if (Input.GetButton("Fire3")) { transformTimer += Time.deltaTime; Color tempColor = AbilityTracker.instance.transformGrayLayer.color; tempColor.a = 0.6f; AbilityTracker.instance.transformGrayLayer.color = tempColor; AbilityTracker.instance.transformGrayLayer.fillAmount = transformTimer / transformTime; if (transformTimer >= transformTime) { standingPlayer.SetActive(false); transformedPlayer.SetActive(true); transformed = true; transformCoolTimer = transformCooldown; transformCooled = false; AbilityTracker.instance.AbilityUpdate(); transformTimer = 0; } } else if (Input.GetButtonUp("Fire3")) { transformTimer = 0; Color tempColor = AbilityTracker.instance.transformGrayLayer.color; tempColor.a = 0f; AbilityTracker.instance.transformGrayLayer.color = tempColor; } } if (transformCoolTimer > 0 && !transformCooled && AbilityTracker.instance.transformAbilityUnlocked) { transformCoolTimer -= Time.deltaTime; Color tempColor = AbilityTracker.instance.transformGrayLayer.color; tempColor.a = 0.6f; AbilityTracker.instance.transformGrayLayer.color = tempColor; AbilityTracker.instance.transformGrayLayer.fillAmount = 0 + transformCoolTimer / transformCooldown; if (transformCoolTimer
Enemy Control
public class EnemyController : MonoBehaviour { public EnemyType type; public Transform[] patrolPoints; private int curPoint; public float moveSpeed; public float turnSpeed; public float waitAtPoint; private float waitCounter; public float jumpForce; public Rigidbody2D theRB; public bool isGrounded = true; public float wallTime; private float wallCounter; public bool isWalled; private bool walled; public Transform groundPoint; public Transform wallPoint; public LayerMask groundLayer; public Animator anim; public bool enemyDetect; public GameObject playerChar; private void Start() { waitCounter = waitAtPoint; wallCounter = wallTime; foreach (Transform pPoint in patrolPoints) { pPoint.SetParent(null); } } private void Update() { if (type == EnemyType.Patroller) { if (Mathf.Abs(transform.position.x - patrolPoints[curPoint].position.x) > 0.2f && !enemyDetect) { if (transform.position.x < patrolPoints[curPoint].position.x) { theRB.velocity = new Vector2(moveSpeed, theRB.velocity.y); transform.localScale = new Vector3(-1f, 1f, 1f); } else { theRB.velocity = new Vector2(-moveSpeed, theRB.velocity.y); transform.localScale = new Vector3(1f, 1f, 1f); } walled = Physics2D.OverlapCircle(wallPoint.position, 0.2f, groundLayer); if (walled) { wallCounter -= Time.deltaTime; if (wallCounter <= 0) { wallCounter = wallTime; isWalled = true; } } else { isWalled = false; wallCounter = wallTime; } isGrounded = Physics2D.OverlapCircle(groundPoint.position, 0.2f, groundLayer); if (transform.position.y < patrolPoints[curPoint].position.y - 0.3f && theRB.velocity.y < 0.1f && isGrounded) { theRB.velocity = new Vector2(theRB.velocity.x, jumpForce); isGrounded = false; } else if (isGrounded && isWalled) { theRB.velocity = new Vector2(theRB.velocity.x, jumpForce); isGrounded = false; isWalled = false; } } else if (Mathf.Abs(transform.position.x - patrolPoints[curPoint].position.x) < 0.2f && !enemyDetect) { theRB.velocity = new Vector2(0f, theRB.velocity.y); waitCounter -= Time.deltaTime; if (waitCounter = patrolPoints.Length) { curPoint = 0; } } } else if (enemyDetect && playerChar != null) { if (transform.position.x < playerChar.transform.position.x) { theRB.velocity = new Vector2(moveSpeed, theRB.velocity.y); transform.localScale = new Vector3(-1f, 1f, 1f); } else { theRB.velocity = new Vector2(-moveSpeed, theRB.velocity.y); transform.localScale = new Vector3(1f, 1f, 1f); } isGrounded = Physics2D.OverlapCircle(groundPoint.position, 0.2f, groundLayer); if (transform.position.y < playerChar.transform.position.y - 0.6f && theRB.velocity.y 0.2f && !enemyDetect) { Vector2 direction = (transform.position - patrolPoints[curPoint].transform.position).normalized; float angle = Mathf.Atan2(direction.y, direction.x) * Mathf.Rad2Deg; Quaternion targetRot = Quaternion.AngleAxis(angle, Vector3.forward); transform.rotation = Quaternion.Slerp(transform.rotation, targetRot, turnSpeed * Time.deltaTime); theRB.velocity = new Vector2(-direction.x * moveSpeed, -direction.y * moveSpeed) * Time.deltaTime; walled = Physics2D.OverlapCircle(wallPoint.position, 0.2f, groundLayer); if (walled) { wallCounter -= Time.deltaTime; if (wallCounter <= 0) { wallCounter = wallTime; isWalled = true; } } else { isWalled = false; wallCounter = wallTime; } isGrounded = Physics2D.OverlapCircle(groundPoint.position, 0.2f, groundLayer); if (transform.position.y < patrolPoints[curPoint].position.y - 0.3f && theRB.velocity.y < 0.1f && isGrounded) { theRB.velocity = new Vector2(theRB.velocity.x, jumpForce); isGrounded = false; } else if (isGrounded && isWalled) { theRB.velocity = new Vector2(theRB.velocity.x, jumpForce); isGrounded = false; isWalled = false; } } else if (Mathf.Abs(transform.position.x - patrolPoints[curPoint].position.x) < 0.2f && !enemyDetect) { theRB.velocity = new Vector2(0f, theRB.velocity.y); waitCounter -= Time.deltaTime; if (waitCounter = patrolPoints.Length) { curPoint = 0; } } } else if (enemyDetect && playerChar != null) { Vector2 direction = (transform.position - playerChar.transform.position).normalized; float angle = Mathf.Atan2(direction.y, direction.x) * Mathf.Rad2Deg; Quaternion targetRot = Quaternion.AngleAxis(angle, Vector3.forward); transform.rotation = Quaternion.Slerp(transform.rotation, targetRot, turnSpeed * Time.deltaTime); //transform.position += (-transform.right * moveSpeed) * Time.deltaTime; theRB.velocity = new Vector2(-direction.x * moveSpeed, -direction.y * moveSpeed) * Time.deltaTime; walled = Physics2D.OverlapCircle(wallPoint.position, 1.5f, groundLayer); if (walled) { wallCounter -= Time.deltaTime; if (wallCounter
Game Control
public class GameManager : MonoBehaviour { public int curTurn; public bool placingBuilding; public BuildingType curSelectedBuilding; [Header("Camera Points")] public Transform cameraPointA; public Transform cameraPointB; public bool cameraA; public Camera mainCamera; public TextMeshProUGUI dungeonText; [Header("BuildingList")] public GameObject buildList; public bool hideList; public TextMeshProUGUI buildText; [Header("Current Resources")] public int curFood; public int curMetal; public int curOxygen; public int curEnergy; public int curScience; public int curPopulation; public int curCorePoints; public int curBuildings; public int curMaxBuildings; [Header("Round Resource Increase")] public int foodPerTurn; public int metalPerTurn; public int oxygenPerTurn; public int energyPerTurn; public int sciencePerTurn; public int corePointsPerTurn; [Header("Population Changes")] public int populationBirth; public int populationDeath; public int totalPopulationChange; [HideInInspector] public float smallCycleTime, greatCycleTime, currentSmallCycleTime, currentGreatCycleTime; [HideInInspector] public bool smallCycleStart, greatCycleStart, greatCycleDone; public static GameManager instance; private void Awake() { if (instance != null) { Destroy(gameObject); } else { instance = this; DontDestroyOnLoad(gameObject); } smallCycleTime = 5; greatCycleTime = smallCycleTime * 4; smallCycleStart = true; greatCycleStart = true; greatCycleDone = true; } private void Start() { Ui.instance.UpdateResourcetext(); cameraA = true; hideList = true; buildList.SetActive(false); } public void Update() { if (smallCycleStart) { currentSmallCycleTime += Time.deltaTime; if (currentSmallCycleTime >= smallCycleTime) { EndTurn(); currentSmallCycleTime = 0; } } if (greatCycleStart) { currentGreatCycleTime += Time.deltaTime; if (currentGreatCycleTime >= greatCycleTime) { greatCycleDone = true; greatCycleStart = false; currentGreatCycleTime = 0; } } } public void EndTurn() { curFood += foodPerTurn; curMetal += metalPerTurn; curOxygen += oxygenPerTurn; curEnergy += energyPerTurn; curScience += sciencePerTurn; curCorePoints += corePointsPerTurn; Ui.instance.UpdateResourcetext(); curTurn++; if (greatCycleDone) { Ui.instance.ToggleBuildingButtons(true); Map.instance.EnableUsableTiles(); greatCycleDone = false; greatCycleStart = true; currentGreatCycleTime = 0; } } public void SetPlacingBuilding(BuildingType buildingType) { placingBuilding = true; curSelectedBuilding = buildingType; Debug.Log("select" + curSelectedBuilding); } public void ChangeView() { if (cameraA) { mainCamera.transform.position = cameraPointB.position; dungeonText.text = "Village"; cameraA = false; } else { mainCamera.transform.position = cameraPointA.position; dungeonText.text = "Dungeon"; cameraA = true; } } public void OpenBuildList() { if (hideList) { buildList.SetActive(true); buildText.text = "Close"; hideList = false; } else { buildList.SetActive(false); buildText.text = "Building List"; hideList = true; } } public void OnCreatedNewBuilding(Building building) { if (building.doesProduce) { switch (building.productionResource) { case ResourceType.Food: foodPerTurn += building.productionPerTurn; break; case ResourceType.Material: metalPerTurn += building.productionPerTurn; break; case ResourceType.Water: oxygenPerTurn += building.productionPerTurn; break; case ResourceType.Energy: energyPerTurn += building.productionPerTurn; break; } } if (building.upkeepCost) { switch (building.upkeepResource) { case ResourceType.Food: foodPerTurn -= building.costPerTurn; break; case ResourceType.Material: metalPerTurn -= building.costPerTurn; break; case ResourceType.Water: oxygenPerTurn -= building.costPerTurn; break; case ResourceType.Energy: energyPerTurn -= building.costPerTurn; break; } } placingBuilding = false; Ui.instance.UpdateResourcetext(); } }
2D Strat Map
public class Map : MonoBehaviour { public List tiles = new List(); public List buildings = new List(); public float tileSize; public List buildingPrefabs = new List(); public static Map instance; private void Awake() { if (instance != null) { Destroy(gameObject); } else { instance = this; DontDestroyOnLoad(gameObject); } } void Start() { EnableUsableTiles(); if (buildings.Count > 0) { Building building = buildings[0]; if (building.type == BuildingType.Base) { GameManager.instance.curFood = 10; GameManager.instance.curEnergy = 10; GameManager.instance.curMetal = 10; GameManager.instance.curOxygen = 10; if (building.doesProduce) { switch (building.productionResource) { case ResourceType.Food: GameManager.instance.foodPerTurn += building.productionPerTurn; break; case ResourceType.Material: GameManager.instance.metalPerTurn += building.productionPerTurn; break; case ResourceType.Water: GameManager.instance.oxygenPerTurn += building.productionPerTurn; break; case ResourceType.Energy: GameManager.instance.energyPerTurn += building.productionPerTurn; break; } } if (building.upkeepCost) { switch (building.upkeepResource) { case ResourceType.Food: GameManager.instance.foodPerTurn -= building.costPerTurn; break; case ResourceType.Material: GameManager.instance.metalPerTurn -= building.costPerTurn; break; case ResourceType.Water: GameManager.instance.oxygenPerTurn -= building.costPerTurn; break; case ResourceType.Energy: GameManager.instance.energyPerTurn -= building.costPerTurn; break; } } if (building.providesPop) { switch (building.populationResource) { case ResourceType.Population: GameManager.instance.curPopulation += building.totalChange; break; } } else if (building.consumePop) { switch (building.populationResource) { case ResourceType.Population: GameManager.instance.curPopulation -= building.totalChange; break; } } Ui.instance.UpdateResourcetext(); } } } public void EnableUsableTiles() { foreach (Building building in buildings) { Tile northTile = GetTileAtPosition(building.transform.position + new Vector3(-tileSize / 2, tileSize / 1.2f, 0)); Tile eastTile = GetTileAtPosition(building.transform.position + new Vector3(tileSize, 0, 0)); Tile westTile = GetTileAtPosition(building.transform.position + new Vector3(-tileSize, 0, 0)); Tile southTile = GetTileAtPosition(building.transform.position + new Vector3(-tileSize / 2, -tileSize / 1.2f, 0)); Tile north1Tile = GetTileAtPosition(building.transform.position + new Vector3(tileSize / 2, tileSize / 1.2f, 0)); Tile south1Tile = GetTileAtPosition(building.transform.position + new Vector3(tileSize / 2, -tileSize / 1.2f, 0)); northTile?.ToggleHighlight(true); southTile?.ToggleHighlight(true); north1Tile?.ToggleHighlight(true); south1Tile?.ToggleHighlight(true); eastTile?.ToggleHighlight(true); westTile?.ToggleHighlight(true); } } public void DisableUsableTiles() { foreach (Tile tile in tiles) { tile.ToggleHighlight(false); } } public void CreateNewBuilding(BuildingType type, Vector3 position) { Debug.Log(type); Building prefabToSpawn = buildingPrefabs.Find(x => x.type == type); Debug.Log("spawn" + prefabToSpawn); GameObject buildingObj = Instantiate(prefabToSpawn.gameObject, position, Quaternion.identity); buildings.Add(buildingObj.GetComponent()); GetTileAtPosition(position).hasBuilding = true; DisableUsableTiles(); GameManager.instance.OnCreatedNewBuilding(prefabToSpawn); GameManager.instance.greatCycleDone = false; GameManager.instance.greatCycleStart = true; } Tile GetTileAtPosition(Vector3 pos) { return tiles.Find(x => x.CanBeHighlighted(pos)); } }
Interface Control
public class InteractionMng : MonoBehaviour { public float checkRate = 0.05f; private float lastCheckTime; public float maxCheckDistance; public LayerMask layerMask; private GameObject curInteractGO; private IInteractable curInteractable; public TextMeshProUGUI promptText; public GameObject promptBcg; private Camera cam; private void Start() { cam = Camera.main; } private void Update() { if(Time.time - lastCheckTime > checkRate) { lastCheckTime = Time.time; Ray ray = cam.ScreenPointToRay(new Vector3(Screen.width/2, Screen.height/2, 0)); RaycastHit hit; if(Physics.Raycast(ray, out hit, maxCheckDistance, layerMask)) { if(hit.collider.gameObject != curInteractGO) { curInteractGO = hit.collider.gameObject; curInteractable = hit.collider.GetComponent(); SetPromptText(); } } else { curInteractGO = null; curInteractable = null; promptText.gameObject.SetActive(false); promptBcg.SetActive(false); } } } private void SetPromptText() { promptText.gameObject.SetActive(true); promptBcg.SetActive(true); promptText.text = string.Format("[E] {0}", curInteractable.GetInteractPrompt()); } public void OnInteractInput(InputAction.CallbackContext context) { if(context.phase == InputActionPhase.Started && curInteractable != null) { curInteractable.OnInteract(); curInteractGO = null; curInteractable = null; promptText.gameObject.SetActive(false); promptBcg.SetActive(false); } } } public interface IInteractable { string GetInteractPrompt(); void OnInteract(); }
Inventory System
public class Inventory : MonoBehaviour { public ItemSlotUi[] uiSlots; public ItemSlot[] slots; public GameObject inventoryWindow; public Transform dropPosition; [Header("Selected Item")] public ItemSlot selectedItem; private int selectedItemIndex; public TextMeshProUGUI selectedItemName; public TextMeshProUGUI selectedItemDescription; public TextMeshProUGUI selectedItemStatName; public TextMeshProUGUI selectedItemStatValue; public GameObject useButton; public GameObject equipButton; public GameObject unEquipButton; public GameObject dropButton; private int curEquipIndex; private PlayerController controller; private PlayerStats needs; public UnityEvent onOpenInventory; public UnityEvent onCloseInventory; public static Inventory instance; private void Awake() { instance = this; controller = GetComponent(); needs = GetComponent(); } private void Start() { inventoryWindow.SetActive(false); slots = new ItemSlot[uiSlots.Length]; for (int x = 0; x < slots.Length; x++) { slots[x] = new ItemSlot(); uiSlots[x].index = x; uiSlots[x].clear(); } ClearSelectedItemWindow(); } public void OnInventoryButton(InputAction.CallbackContext context) { if(context.phase == InputActionPhase.Started) { Toggle(); } } public void Toggle() { if(inventoryWindow.activeInHierarchy) { inventoryWindow.SetActive(false); onCloseInventory.Invoke(); controller.ToggleCursor(false); } else { inventoryWindow.SetActive(true); onOpenInventory.Invoke(); ClearSelectedItemWindow(); controller.ToggleCursor(true); } } public bool IsOpen() { return inventoryWindow.activeInHierarchy; } public void AddItem (ItemData item) { if(item.canStack) { ItemSlot slotToStackTo = GetItemStack(item); if(slotToStackTo != null) { slotToStackTo.quantity++; UpdateUi(); return; } } ItemSlot emptySlot = GetEmptySlot(); if(emptySlot != null) { emptySlot.item = item; emptySlot.quantity = 1; UpdateUi(); return; } ThrowItem(item); } public void ThrowItem(ItemData item) { Instantiate(item.dropPrefab, new Vector3(dropPosition.position.x, dropPosition.position.y + 0.5f, dropPosition.position.z), Quaternion.Euler(Vector3.one * Random.value * 360.0f)); } public void UpdateUi() { for(int x =0; x < slots.Length; x++) { if(slots[x].item != null) { uiSlots[x].set(slots[x]); } else { uiSlots[x].clear(); } } } ItemSlot GetItemStack (ItemData item) { for (int x = 0; x < slots.Length; x++) { if(slots[x].item == item && slots[x].quantity < item.maxStackAmount) { return slots[x]; } } return null; } ItemSlot GetEmptySlot() { for (int x = 0; x < slots.Length; x++) { if(slots[x].item == null) { return slots[x]; } } return null; } public void SelectItem(int index) { if (slots[index].item == null) return; selectedItem = slots[index]; selectedItemIndex = index; selectedItemName.text = selectedItem.item.displayName; selectedItemDescription.text = selectedItem.item.description; selectedItemStatName.text = string.Empty; selectedItemStatValue.text = string.Empty; for(int x = 0; x < selectedItem.item.consumables.Length; x++) { selectedItemStatName.text += selectedItem.item.consumables[x].type.ToString() + ": " + "\n"; selectedItemStatValue.text += selectedItem.item.consumables[x].value.ToString() + "\n"; } useButton.SetActive(selectedItem.item.type == ItemType.Food || selectedItem.item.type == ItemType.Drinks); equipButton.SetActive(selectedItem.item.type == ItemType.Equipable && !uiSlots[index].equipped); unEquipButton.SetActive(selectedItem.item.type == ItemType.Equipable && uiSlots[index].equipped); dropButton.SetActive(true); } void ClearSelectedItemWindow() { selectedItem = null; selectedItemName.text = string.Empty; selectedItemDescription.text = string.Empty; selectedItemStatName.text = string.Empty; selectedItemStatValue.text = string.Empty; useButton.SetActive(false); dropButton.SetActive(false); equipButton.SetActive(false); unEquipButton.SetActive(false); } public void OnUseButton() { if(selectedItem.item.type == ItemType.Food || selectedItem.item.type == ItemType.Drinks) { for (int x = 0; x < selectedItem.item.consumables.Length; x++) { switch(selectedItem.item.consumables[x].type) { case ConsumableType.Health: needs.heal(selectedItem.item.consumables[x].value); break; case ConsumableType.Thirst: needs.drink(selectedItem.item.consumables[x].value); break; case ConsumableType.Hunger: needs.eat(selectedItem.item.consumables[x].value); break; case ConsumableType.Sleep: needs.rest(selectedItem.item.consumables[x].value); break; } } } RemoveSelectedItem(); } public void OnEquipButton() { if (uiSlots[curEquipIndex].equipped) UnEquip(curEquipIndex); uiSlots[selectedItemIndex].equipped = true; curEquipIndex = selectedItemIndex; EquipMng.instance.EquipNew(selectedItem.item); UpdateUi(); SelectItem(selectedItemIndex); } public void UnEquip (int index) { uiSlots[index].equipped = false; EquipMng.instance.Unequip(); UpdateUi(); if (selectedItemIndex == index) SelectItem(index); } public void OnUnEquipButton() { UnEquip(selectedItemIndex); } public void OnDropButton() { ThrowItem(selectedItem.item); RemoveSelectedItem(); } void RemoveSelectedItem() { selectedItem.quantity--; if(selectedItem.quantity == 0) { if(uiSlots[selectedItemIndex].equipped == true) { UnEquip(selectedItemIndex); } selectedItem.item = null; ClearSelectedItemWindow(); } UpdateUi(); } public void RemoveItem (ItemData item) { for(int i = 0; i < slots.Length; i ++) { if(slots[i].item == item) { slots[i].quantity--; if (slots[i].quantity == 0) { if (uiSlots[i].equipped == true) UnEquip(i); slots[i].item = null; ClearSelectedItemWindow(); } UpdateUi(); return; } } } public bool HasItems (ItemData item, int quantity) { int amount = 0; for(int i =0; i = quantity) return true; } return false; } } public class ItemSlot { public ItemData item; public int quantity; }
AI State Control
public enum AIType { Passive, Scared, Aggressive } public enum AIState { Idle, Wandering, Attacking, Fleeing } public class NPC : MonoBehaviour, Idamagable { [Header("Stats")] public int health; public float walkSpeed; public float runSpeed; public ItemData[] dropOnDeath; [Header("AI")] public AIType aiType; private AIState aiState; public float detectDistance; public float safeDistance; [Header("Wandering")] public float minWanderDistance; public float maxWanderDistance; public float minWanderWaitTime; public float maxWanderWaitTime; [Header("Combat")] public int damage; public float attackRate; private float lastAttackTime; public float attackDistance; private float playerDistance; private NavMeshAgent agent; private Animator anim; private SkinnedMeshRenderer[] meshRenderers; public LayerMask layer; void Awake () { agent = GetComponent(); anim = GetComponentInChildren(); meshRenderers = GetComponentsInChildren(); } void Start () { SetState(AIState.Wandering); } void Update () { playerDistance = Vector3.Distance(transform.position, PlayerController.instance.transform.position); anim.SetBool("Moving", aiState != AIState.Idle); switch(aiState) { case AIState.Idle: PassiveUpdate(); break; case AIState.Wandering: PassiveUpdate(); break; case AIState.Attacking: AttackingUpdate(); break; case AIState.Fleeing: FleeingUpdate(); break; } } void PassiveUpdate () { if(aiState == AIState.Wandering && agent.remainingDistance < 0.1f) { SetState(AIState.Idle); Invoke("WanderToNewLocation", Random.Range(minWanderWaitTime, maxWanderWaitTime)); } if(aiType == AIType.Aggressive && playerDistance < detectDistance) { SetState(AIState.Attacking); } else if(aiType == AIType.Scared && playerDistance attackDistance) { agent.isStopped = false; agent.SetDestination(PlayerController.instance.transform.position); } else { agent.isStopped = true; if(Time.time - lastAttackTime > attackRate) { lastAttackTime = Time.time; PlayerController.instance.GetComponent().takeDamage(damage); anim.SetTrigger("Attack"); } } } void FleeingUpdate () { if(playerDistance < safeDistance && agent.remainingDistance safeDistance) { SetState(AIState.Wandering); } } void SetState (AIState newState) { aiState = newState; switch(aiState) { case AIState.Idle: { agent.speed = walkSpeed; agent.isStopped = true; break; } case AIState.Wandering: { agent.speed = walkSpeed; agent.isStopped = false; break; } case AIState.Attacking: { agent.speed = runSpeed; agent.isStopped = false; break; } case AIState.Fleeing: { agent.speed = runSpeed; agent.isStopped = false; break; } } } void WanderToNewLocation () { if(aiState != AIState.Idle) return; SetState(AIState.Wandering); agent.SetDestination(GetWanderLocation()); } Vector3 GetWanderLocation () { NavMeshHit hit; NavMesh.SamplePosition(transform.position + (Random.onUnitSphere * Random.Range(minWanderDistance, maxWanderDistance)), out hit, maxWanderDistance, NavMesh.AllAreas); int i = 0; while(Vector3.Distance(transform.position, hit.position) 90 || playerDistance < safeDistance) { NavMesh.SamplePosition(transform.position + (Random.onUnitSphere * safeDistance), out hit, safeDistance, NavMesh.AllAreas); i++; if(i == 30) break; } return hit.position; } float GetDestinationAngle (Vector3 targetPos) { return Vector3.Angle(transform.position - PlayerController.instance.transform.position, transform.position + targetPos); } public void takeDamage(int damageAmount) { health -= damageAmount; if(health <= 0) Die(); StartCoroutine(DamageFlash()); if(aiType == AIType.Passive) SetState(AIState.Fleeing); } void Die () { for(int x = 0; x < dropOnDeath.Length; x++) { Instantiate(dropOnDeath[x].dropPrefab, transform.position, Quaternion.identity); } Destroy(gameObject); } IEnumerator DamageFlash () { for(int x = 0; x < meshRenderers.Length; x++) meshRenderers[x].material.color = new Color(1.0f, 0.6f, 0.6f); yield return new WaitForSeconds(0.1f); for(int x = 0; x
Terrain Tile Generator
public enum TerrainVisualization { Height, Heat, Moisture, Biome } public class TileGenerator : MonoBehaviour { [Header("Parameters")] public int noiseSampleSize; public float scale; public int textureResolution = 1; public float maxHeight = 1.0f; public TerrainVisualization visualizationType; [HideInInspector] public Vector2 offset; [Header("Terrain Types")] public TerrainType[] heightTerrainTypes; public TerrainType[] heatTerrainTypes; public TerrainType[] moistureTerrainTypes; [Header("Waves")] public Wave[] waves; public Wave[] heatWaves; public Wave[] moistureWaves; private MeshRenderer tileMeshRender; private MeshFilter tileMeshFilter; private MeshCollider tileMeshCollider; private MeshGenerator meshGenerator; private MapGenerator mapGenerator; private TerrainData[,] dataMap; public bool randomGen; private void Awake() { randomGen = SettingsData.instance.randomGen; if (randomGen) { int length = waves.Length; for (int x = 0; x < length; x++) { waves[x].amplitude += SettingsData.instance.amplitudeAdd; waves[x].seed += SettingsData.instance.seedAdd; waves[x].frequency += SettingsData.instance.frequencyAdd; } int heatLength = heatWaves.Length; for (int x = 0; x < heatLength; x++) { heatWaves[x].amplitude += SettingsData.instance.amplitudeAdd; heatWaves[x].seed += SettingsData.instance.seedAdd; heatWaves[x].frequency += SettingsData.instance.frequencyAdd; } int moistureLength = moistureWaves.Length; for (int x = 0; x < moistureLength; x++) { moistureWaves[x].amplitude += SettingsData.instance.amplitudeAdd; moistureWaves[x].seed += SettingsData.instance.seedAdd; moistureWaves[x].frequency += SettingsData.instance.frequencyAdd; } } } private void Start() { tileMeshCollider = GetComponent(); tileMeshFilter = GetComponent(); tileMeshRender = GetComponent(); meshGenerator = GetComponent(); mapGenerator = FindObjectOfType(); GenerateTile(); } private void GenerateTile() { float[,] heightMap = NoiseGenerator.GenerateNoisemap(noiseSampleSize, scale, waves, offset, randomGen); float[,] hdHeightMap = NoiseGenerator.GenerateNoisemap(noiseSampleSize - 1, scale, waves, offset, randomGen, textureResolution); Vector3[] verts = tileMeshFilter.mesh.vertices; for (int x = 0; x < noiseSampleSize; x++) { for (int z = 0; z < noiseSampleSize; z++) { int index = (x * noiseSampleSize) + z; verts[index].y = heightMap[x, z] * maxHeight; } } tileMeshFilter.mesh.vertices = verts; tileMeshFilter.mesh.RecalculateBounds(); tileMeshFilter.mesh.RecalculateNormals(); tileMeshCollider.sharedMesh = tileMeshFilter.mesh; Texture2D heightMapTexture = TextureBuilder.BuildTexture(heightMap, heightTerrainTypes); float[,] heatMap = GenerateHeatMap(heightMap); float[,] moistureMap = GenerateMoistureMap(heightMap); TerrainType[,] heatTerrainTypeMap = TextureBuilder.CreateTerrainTypeMap(heatMap, heatTerrainTypes); TerrainType[,] moistureTerrainTypeMap = TextureBuilder.CreateTerrainTypeMap(moistureMap, moistureTerrainTypes); Texture2D biomeTexture = BiomeBuilder.instance.BuildTexture(heatTerrainTypeMap, moistureTerrainTypeMap); switch (visualizationType) { case TerrainVisualization.Height: tileMeshRender.material.mainTexture = heightMapTexture; break; case TerrainVisualization.Heat: tileMeshRender.material.mainTexture = TextureBuilder.BuildTexture(heatMap, heatTerrainTypes); break; case TerrainVisualization.Moisture: tileMeshRender.material.mainTexture = TextureBuilder.BuildTexture(moistureMap, moistureTerrainTypes); break; case TerrainVisualization.Biome: tileMeshRender.material.mainTexture = BiomeBuilder.instance.BuildFinalTexture(biomeTexture, heightMap, heightTerrainTypes); break; } CreateDataMap(heatTerrainTypeMap, moistureTerrainTypeMap); TreeSpawner.instance.Spawn(dataMap, heightMap, maxHeight); } public void CreateDataMap(TerrainType[,] heatTerrainTypeMap, TerrainType[,] moistureTerrainTypeMap) { dataMap = new TerrainData[noiseSampleSize, noiseSampleSize]; Vector3[] verts = tileMeshFilter.mesh.vertices; for (int x = 0; x < noiseSampleSize; x++) { for (int z = 0; z < noiseSampleSize; z++) { TerrainData data = new TerrainData(); data.position = transform.position + verts[(x * noiseSampleSize) + z]; data.heatTerrainType = heatTerrainTypeMap[x, z]; data.moistureTerrainType = moistureTerrainTypeMap[x, z]; data.biome = BiomeBuilder.instance.GetBiome(data.heatTerrainType, data.moistureTerrainType); dataMap[x, z] = data; } } } float[,] GenerateHeatMap(float[,] heightMap) { float[,] uniformHeatMap = NoiseGenerator.GenerateUniformNoiseMap(noiseSampleSize, transform.position.z * (noiseSampleSize / meshGenerator.xSize), (noiseSampleSize / 2 * mapGenerator.numX) + 1); float[,] randomHeatMap = NoiseGenerator.GenerateNoisemap(noiseSampleSize, scale, heatWaves, offset, randomGen); float[,] heatMap = new float[noiseSampleSize, noiseSampleSize]; for (int x = 0; x < noiseSampleSize; x++) { for (int z = 0; z < noiseSampleSize; z++) { heatMap[x, z] = randomHeatMap[x, z] * uniformHeatMap[x, z]; heatMap[x, z] += 0.5f * heightMap[x, z]; heatMap[x, z] = Mathf.Clamp(heatMap[x, z], 0.0f, 0.99f); } } return heatMap; } float[,] GenerateMoistureMap(float[,] heightMap) { float[,] moistureMap = NoiseGenerator.GenerateNoisemap(noiseSampleSize, scale, moistureWaves, offset, randomGen); for (int x = 0; x < noiseSampleSize; x++) { for (int z = 0; z
Biome Generator
public enum BiomeType { Desert, Tundra, Savanna, Forest, RainForest } public class BiomeBuilder : MonoBehaviour { public Biome[] biomes; public BiomeRow[] tableRows; public static BiomeBuilder instance; private void Awake() { instance = this; } public Texture2D BuildTexture (TerrainType[,] heatMapTypes, TerrainType[,] moistureMapTypes) { int size = heatMapTypes.GetLength(0); Color[] pixels = new Color[size * size]; for(int x = 0; x < size; x ++) { for(int z = 0; z < size; z ++) { int index = (x * size) + z; int heatMapIndex = heatMapTypes[x, z].index; int moistureMapIndex = moistureMapTypes[x, z].index; Biome biome = null; foreach(Biome b in biomes) { if(b.type == tableRows[moistureMapIndex].tableColums[heatMapIndex]) { biome = b; break; } } pixels[index] = biome.color; } } Texture2D texture = new Texture2D(size, size); texture.wrapMode = TextureWrapMode.Clamp; texture.filterMode = FilterMode.Bilinear; texture.SetPixels(pixels); texture.Apply(); return texture; } public Texture2D BuildFinalTexture(Texture2D biomeTexture, float[,] noiseMap, TerrainType[] terrainTypes) { Color[] pixels = new Color[noiseMap.Length]; int pixelLength = noiseMap.GetLength(0); for (int x = 0; x < pixelLength; x++) { for (int z = 0; z < pixelLength; z++) { int index = (x * pixelLength) + z; for (int t = 0; t < terrainTypes.Length; t++) { if (noiseMap[x, z] < terrainTypes[t].threshhold & noiseMap[x, z]
Terrain Texture Painter
public class TextureBuilder { public static Texture2D BuildTexture (float[,] noiseMap, TerrainType[] terrainTypes) { Color[] pixels = new Color[noiseMap.Length]; int pixelLength = noiseMap.GetLength(0); for(int x = 0; x < pixelLength; x++) { for(int z = 0; z < pixelLength; z++) { int index = (x * pixelLength) + z; for(int t = 0; t < terrainTypes.Length; t++) { if(noiseMap[x,z] < terrainTypes[t].threshhold) { float minVal = t == 0 ? 0 : terrainTypes[t - 1].threshhold; float maxVal = terrainTypes[t].threshhold; pixels[index] = terrainTypes[t].colorGradient.Evaluate(1.0f - (maxVal - noiseMap[x, z]) / (maxVal - minVal)); break; } } } } Texture2D texture = new Texture2D(pixelLength, pixelLength); texture.wrapMode = TextureWrapMode.Clamp; texture.filterMode = FilterMode.Bilinear; texture.SetPixels(pixels); texture.Apply(); return texture; } public static TerrainType[,] CreateTerrainTypeMap (float[,] noiseMap, TerrainType[] terrainTypes) { int size = noiseMap.GetLength(0); TerrainType[,] outputMap = new TerrainType[size, size]; for(int x = 0; x < size; x ++) { for(int z = 0; z < size; z ++) { for(int t = 0; t < terrainTypes.Length; t ++) { if(noiseMap[x, z]
Idle Points System
public class DungeonPoints : MonoBehaviour { public static DungeonPoints instance; public float gold; public float perClick; public float idleGold; public float goldMulti; public TextMeshProUGUI goldCount; public TextMeshProUGUI clickCount; public TextMeshProUGUI idleCount; public AudioSource click; public List idleClickLastTime = new List(); private void Awake() { instance = this; gold = 0; perClick = 1; idleGold = 0; goldMulti = 1; } private void Update() { for (int i = 0; i = 1.0f) { idleClickLastTime[i] = Time.time; DungeonPoints.instance.PerClick(); } } } public void PerClick() { gold = gold + (perClick * goldMulti); click.PlayOneShot(click.clip); goldCount.text = gold.ToString("g1"); } public void perFloor() { gold = gold + ((perClick * goldMulti) * (Floors.instance.slimeFloors.Count + Floors.instance.ratFloors.Count) * 5); goldCount.text = gold.ToString("g1"); } public void RemoveGold(float amount) { gold = gold - amount; } }
Ability Tracker System
public class AbilityTracker : MonoBehaviour { public bool bombAbilityUnlocked; public Image bombGrayLayer; public Image bombIcon; public Sprite bombSprite; public Sprite basicSprite; public bool dashAbilityUnlocked; public Image dashGrayLayer; public bool transformAbilityUnlocked; public Image transformGrayLayer; public bool doubleJumpAbilityUnlocked; public float fadeSpeed; public bool fadeToBlack, fadeFromBlack; public PlayerController character; public string unlockedMessage; public TMP_Text unlockText; public static AbilityTracker instance; private void Awake() { if (instance != null) { Destroy(gameObject); } else { instance = this; DontDestroyOnLoad(gameObject); } } private void Start() { AbilityUpdate(); } private void Update() { } public void AbilityUpdate() { if (!PlayerController.instance.transformed) { Color tempColor = bombGrayLayer.color; tempColor.a = 0f; bombGrayLayer.color = tempColor; bombIcon.sprite = basicSprite; } else if (PlayerController.instance.transformed && !bombAbilityUnlocked) { Color tempColor = bombGrayLayer.color; tempColor.a = 0.6f; bombGrayLayer.color = tempColor; bombIcon.sprite = bombSprite; } if (bombAbilityUnlocked) { character.bombCooled = true; Color tempColor = bombGrayLayer.color; tempColor.a = 0f; bombGrayLayer.color = tempColor; if (PlayerController.instance.transformed) { bombIcon.sprite = bombSprite; } } if (dashAbilityUnlocked) { character.canDash = true; Color tempColor = dashGrayLayer.color; if (!PlayerController.instance.transformed) { tempColor.a = 0f; dashGrayLayer.color = tempColor; } else { tempColor.a = 0.6f; dashGrayLayer.color = tempColor; dashGrayLayer.fillAmount = 1f; } } if (transformAbilityUnlocked) { Color tempColor = transformGrayLayer.color; tempColor.a = 0f; transformGrayLayer.color = tempColor; } if (doubleJumpAbilityUnlocked) { character.doubleJumpUnlock = true; character.extraJumpCount = 1; } } public void TextUpdate(string text) { unlockText.text = text; unlockText.gameObject.SetActive(true); StartCoroutine(CloseText()); } private IEnumerator CloseText() { yield return new WaitForSeconds(2f); unlockText.gameObject.SetActive(false); } public void FadingtoBlack() { fadeToBlack = true; fadeFromBlack = false; } public void FadingfromBlack() { fadeToBlack = false; fadeFromBlack = true; } }